本文最后更新于:2024年5月18日 下午
组合数据类型(一)
- 字符串的相关操作函数包括但不限于:
len(string)
: 返回字符串的长度。
string[index]
: 获取字符串中索引为index的字符。
string[start:end:step]
: 切片操作,获取字符串的子串。
string.count(substring)
: 统计子串在字符串中出现的次数。
string.find(substring)
: 查找子串在字符串中第一次出现的位置。
string.replace(old, new)
: 替换字符串中的部分内容。
string.upper()
, string.lower()
: 将字符串转换为全大写或全小写。
string.strip()
, string.lstrip()
, string.rstrip()
: 去除字符串两端或指定端的空白字符。
- 列表相关操作函数包括但不限于:
len(list)
: 返回列表的长度。
list.append(element)
: 在列表末尾添加一个元素。
list.extend(iterable)
: 在列表末尾一次性追加另一个可迭代对象的所有元素。
list.insert(index, element)
: 在指定位置插入一个元素。
list.remove(element)
: 移除列表中第一个匹配的元素。
list.pop([index])
: 移除并返回指定位置的元素,默认是移除并返回最后一个元素。
list.index(element)
: 返回列表中第一个匹配元素的索引。
list.count(element)
: 返回元素在列表中出现的次数。
list.sort()
: 对列表进行排序。
list.reverse()
: 将列表中的元素倒序排列。
list.copy()
: 返回列表的浅复制。
- 元组相关操作函数:
len(tuple)
: 返回元组的长度。
tuple.index(element)
: 返回元组中第一个匹配元素的索引。
tuple.count(element)
: 返回元素在元组中出现的次数。
组合数据类型(二)
字典(dict)
字典是一种键值对集合,每个键都是唯一的,并且与一个值相关联。字典的键值对是无序的,键必须是不可变数据类型,如字符串、数字或元组,而值可以是任何数据类型。
创建字典
1 2 3 4 5 6
| empty_dict = {}
fruit_colors = {'apple': 'red', 'banana': 'yellow', 'cherry': 'red'}
square_dict = {i: i**2 for i in range(1, 6)}
|
访问字典
1 2 3 4
| color = fruit_colors['apple']
color = fruit_colors.get('apple', 'default_color')
|
修改字典
1 2 3 4 5 6
| fruit_colors['orange'] = 'orange'
fruit_colors['apple'] = 'green'
del fruit_colors['banana']
|
字典遍历
1 2 3 4 5 6
| for fruit in fruit_colors: print(fruit)
for fruit, color in fruit_colors.items(): print(fruit, color)
|
字典操作函数dict.fromkeys(seq[, value])
fromkeys() 函数用于创建一个新字典,以序列 seq 中元素做字典的键,value 为字典所有键对应的初始值
1 2 3 4 5 6 7
| key_list = ["name", "age", "hobby"] val = "test" d= {} d = d.fromkeys(key_list) print("新字典",d) d = d.fromkeys(key_list, val) print("新字典",d)
|
使用fromkeys方法创建一个新字典,并将key_list中的所有键添加到字典d中。fromkeys方法返回一个新的字典,其中包含指定的键,每个键的值都是None。这是因为fromkeys方法在没有指定值的情况下默认使用None作为所有键的值。再次使用fromkeys方法创建一个新字典,这次指定了val作为所有键的值。这将创建一个新字典,其中包含key_list中的所有键,每个键的值都是字符串”test”。(由于fromkeys方法会创建一个新的字典,所以在第二次调用fromkeys时,实际上覆盖了第一次创建的字典。)
字典的复制与拷贝
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| """复制与浅拷贝的区别:复制相当于引用,即给原字典起了一个别名,而浅拷贝是,对一级目录直接拷贝,而对二级目录只是引用。"""
Alex = [12,'sing'] Thea = {20:'dance'} dict1= {'Alex':Alex,'Thea':Thea} dict_cpl = dict1 dict_cp2 = dict1.copy() print("原始字典dict1: ",dict1) dict1['Alex']= Thea print("修改后的字典dict1: ",dict1) print("字典dict1的复制(与原字典一致): ",dict_cpl) print("浅拷贝字典dict1(不会随着原字典一级目录的改变而改变): ",dict_cp2) Alex_1=Alex.append(10) print(dict_cp2)
|
创建一个名为dict_cpl的变量,并将其赋值为dict1。这种复制方式实际上是创建了dict1的一个引用,而不是一个全新的字典。因此,dict_cpl和dict1指向内存中的同一个对象。创建一个名为dict_cp2的变量,并将其赋值为dict1.copy()。这是使用copy方法进行的浅拷贝,它会创建一个新的字典,但是字典中的可变对象(如列表和字典)仍然是引用。这意味着dict_cp2是dict1的一个独立副本,但是如果修改了dict1中的可变对象,这些修改也会反映在dict_cp2中。
小记
复制(引用)是指向同一个对象的另一个指针,而浅拷贝是创建一个新的对象,但是新对象中的可变元素仍然是原对象中相应元素的引用。在这个例子中,dict_cpl是dict1的引用,而dict_cp2是dict1的浅拷贝。当dict1的’Alex’键被修改时,dict_cpl会显示这个修改,因为它是引用;而dict_cp2不会显示这个修改,因为它是一个新的字典象,但是如果在dict1中修改了Thea字典中的内容,那么dict_cp2中的’Thea’键也会显示这个修改,因为浅拷贝只拷贝一级目录。
集合(set)
集合是一个无序的不重复元素集。集合的元素必须是不可变数据类型,如字符串、数字或元组。集合不支持索引,因为它们是无序的。
创建集合
1 2 3 4 5 6
| empty_set = set()
fruits = {'apple', 'banana', 'cherry'}
squared_set = {i**2 for i in range(1, 6)}
|
集合操作
1 2 3 4 5 6
| fruits.add('orange')
fruits.remove('banana')
is_apple_in_fruits = 'apple' in fruits
|
集合元素的删除
1 2 3 4 5 6 7 8 9 10
| world_tournament_set = {"世界杯排球赛","世界乒乓球锦标赛","世界篮球锦标赛","世界足球锦标赛"} print("世界大赛:", world_tournament_set) world_tournament_set.remove("世界足球锦标赛") print("set.remove()删除元素后:", world_tournament_set) world_tournament_set.discard("世界杯排球赛") print("set.discard()删除元素后:", world_tournament_set) world_tournament_set.pop() print("set.pop()删除元素后:", world_tournament_set) world_tournament_set.clear() print("set.clear()清空元素后:", world_tournament_set)
|
使用remove方法从集合world_tournament_set中删除一个指定的元素”世界足球锦标赛”。使用discard方法尝试从集合world_tournament_set中删除一个指定的元素”世界杯排球赛”。如果该元素不存在,discard方法不会抛出错误。使用pop方法从集合world_tournament_set中随机删除一个元素。由于集合是无序的,所以pop方法会随机选择一个元素进行删除。使用clear方法清空集合world_tournament_set中的所有元素。
运行结果:
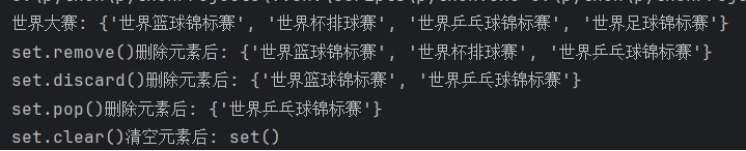
集合遍历
1 2 3
| for fruit in fruits: print(fruit)
|
集合运算
1 2 3 4 5 6 7 8
| union = fruits | {'kiwi', 'mango'}
intersection = fruits & {'apple', 'kiwi'}
difference = fruits - {'apple', 'banana'}
symmetric_difference = fruits ^ {'apple', 'kiwi'}
|